A short step-by-step introduction to NumPy (2024)
Introduction
I’ve been coding in Python for a while now, but it wasn’t until I started using NumPy that I realized its transformative power. Dealing with complex datasets and calculations became so much more manageable. The elegance and efficiency of array operations using NumPy really impressed me. Whether it’s high-speed mathematics or the crunching of big data, NumPy has become an indispensable tool in my arsenal.
Introduction to NumPy and Its Importance
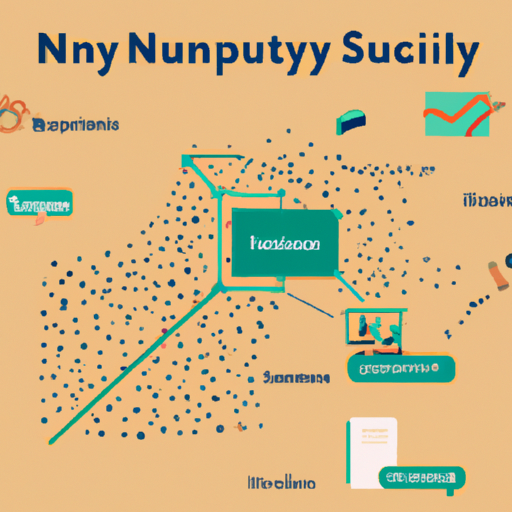
NumPy, which stands for Numerical Python, is the cornerstone library for numerical computing in Python. If you’re doing anything that calls for arrays, you’ll want to know it.
Let’s get straight into it by initializing a NumPy array:
import numpy as np
# Creating a simple NumPy array
= np.array([1, 2, 3, 4, 5])
my_array print(my_array)
This block of code brings NumPy into play, sets up an array, and prints it out—basic but powerful stuff.
You might be wondering, why not just use Python lists? Well, efficiency, for one. NumPy arrays are faster and more compact. Under the hood, NumPy arrays are densely packed in memory due to its fixed types, unlike Python lists. Here’s a quick demonstration:
= list(range(1000))
python_list = np.array(python_list)
numpy_array
%timeit sum(python_list)
%timeit np.sum(numpy_array)
Run this, and you’ll see the NumPy version often smokes the vanilla Python. It’s not just about speed though; it’s about the functionality. NumPy arrays come loaded with operations that would be complex or cumbersome with regular lists.
Mapping math functions over an array is a no-brainer with NumPy:
# Squaring each element
= np.square(my_array)
squared print(squared)
Another crucial advantage NumPy brings is its ability to handle multi-dimensional data. Data in the wild often comes in tables, matrices, or higher-dimensional structures, and NumPy is tuned for that:
# Creating a 2D array (a matrix)
= np.array([[1, 2], [3, 4]])
matrix print(matrix)
To appreciate NumPy’s full potential, imagine working with data where performance really counts—-say, a huge dataset or a computationally intense scientific calculation. Or think about complex operations like matrix multiplication, which is a single, readable line in NumPy:
= np.dot(matrix, matrix)
result print(result)
Launched way back in 2005, NumPy has become a foundational package that serves as a bedrock for the flourishing Python data science ecosystem, including libraries like TensorFlow and Pandas. For credibility’s sake, peer-reviewed research and countless university course pages reference NumPy as an essential tool. You can always find the source code and contribute on its GitHub repository.
To round off this intro, remember that NumPy is vital for anyone aiming to crunch numbers effectively with Python. It’s designed to handle large, multi-dimensional arrays and matrices, along with a sizable collection of high-level mathematical functions to operate on these arrays. I didn’t touch on installation here or delve into the array of more complex operations and functions available in NumPy—that’s covered in the sections that follow.
In the upcoming parts of this larger article, I’ll break down NumPy’s features, from setting up your environment to performing advanced array manipulations and tapping into its power for linear algebra and random number generation. Stick around, and you’ll be streamlining your Python data efforts in no time.
Setting Up Your Environment for NumPy
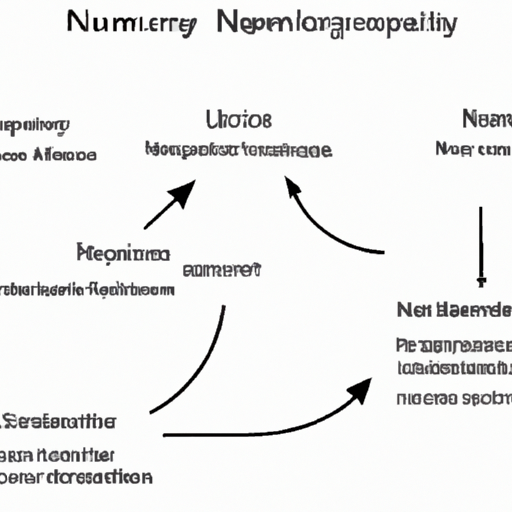
Before diving into the world of NumPy, I need to set up my environment properly. I’ll share the steps I took, hoping it makes the process smoother for you. Whether you’re a beginner or have some experience with Python, you’re going to need NumPy installed to work with arrays efficiently. Here’s how to do it step-by-step.
First up, ensure you have Python installed. You can check by running:
--version python
If Python’s not on your system, head to the official Python website (https://www.python.org/) and grab the installer for your operating system. Throughout this setup, I’m going to use Python 3 since that’s the most recent version.
With Python ready, I’ll set up a virtual environment. This keeps my workspace tidy and my dependencies in check. Using the terminal, I navigate to the project directory and then create a virtual environment with:
-m venv numpy_env python
Now, it’s time to activate the virtual environment. On macOS or Linux, I use:
source numpy_env/bin/activate
For Windows, the command looks like this:
numpy_env\Scripts\activate
Next, I’m going to use pip, Python’s package installer, to set up NumPy. Pip makes installing, upgrading, and removing packages a breeze. Make sure it’s up to date with:
--upgrade pip pip install
With pip updated, installing NumPy is just a command away:
pip install numpy
Niftily, pip downloads and installs NumPy along with any dependencies. After a moment, I verify the installation by firing up Python in interactive mode and importing NumPy:
pythonimport numpy as np
np.__version__
Let’s do a simple array operation to check everything’s working as it should:
= np.array([1, 2, 3, 4, 5])
arr print(arr)
The output confirms NumPy’s array functionality is go. Lastly, I often look for examples or in-depth explanations on Stack Overflow or the NumPy GitHub repository (https://github.com/numpy/numpy) to understand how others solve problems with NumPy.
Finally, if you’re like me and you gravitate towards visual learning, the many tutorials on Jupyter Notebook are a real boon. Get IPython and Jupyter running with:
pip install ipython jupyter
Here’s how to start Jupyter Notebook:
jupyter notebook
Now, a browser window pops up with a slick interface to create and share documents containing live code. It’s an indispensable tool when learning and experimenting.
With all the above setup, I’ve found myself a nice, cozy environment where NumPy and I can spend quality time together. Remember, setting up might seem a bit mundane, but a well-configured environment is the launch pad for all your data adventures with NumPy. Now, my setup is done, and I’m ready for mathematical action!
Basic NumPy Array Operations
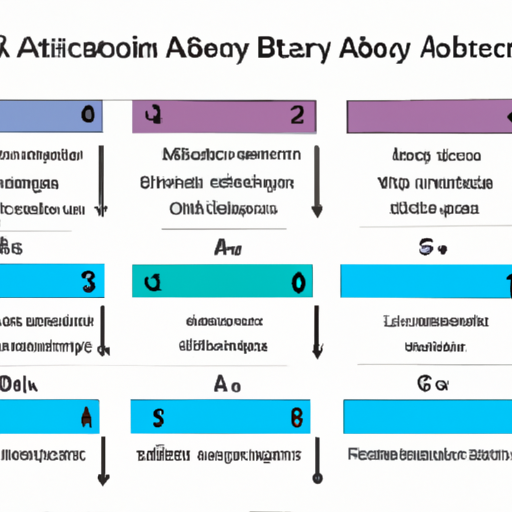
First off, you’ll need an array to work with. NumPy arrays are created using np.array()
. I usually start with something straightforward:
import numpy as np
# Creating a simple array
= np.array([1, 2, 3, 4])
my_array print(my_array)
Once you have an array, one of the most common operations is adding or subtracting a value. You can perform these arithmetic operations element-wise:
# Adding a value to each element
+= 2
my_array print(my_array)
# Subtracting a value from each element
-= 1
my_array print(my_array)
Next up, let’s talk multiplication and division. They’re as intuitive as you might expect:
# Multiplying each element by a value
*= 3
my_array print(my_array)
# Dividing each element by a value
/= 2
my_array print(my_array)
Real talk: operations aren’t just value-based. You can perform these operations on two arrays of the same size, which is incredibly useful:
# Create a new array to operate with
= np.array([5, 6, 7, 8])
another_array
# Element-wise addition of two arrays
= my_array + another_array
result_array print(result_array)
# Element-wise multiplication of two arrays
= my_array * another_array
result_array print(result_array)
I also quickly learned about the aggregation functions in NumPy that save so much time. Functions like np.sum()
, np.mean()
, np.max()
, and np.min()
provide quick insights into your data.
# Sum of all elements in the array
= np.sum(my_array)
sum_of_array print(sum_of_array)
# Mean value of the array elements
= np.mean(my_array)
mean_value print(mean_value)
Need to find the max or min? No sweat:
# Maximum and minimum value in the array
= np.max(my_array)
max_value = np.min(my_array)
min_value print(max_value, min_value)
Arrays aren’t just one-dimensional, of course. You can reshape an array, turn a one-dimensional array into two-dimensional, and execute all the operations I just went through.
# Reshaping the array to a 2x2
= my_array.reshape(2, 2)
reshaped_array print(reshaped_array)
# Multiplying two 2D arrays element-wise
= np.array([[10, 20], [30, 40]])
another_2d_array = reshaped_array * another_2d_array
multiplied_matrix print(multiplied_matrix)
One pro tip: keep an eye on the shape of your arrays. Operations on two arrays can only happen if the arrays are broadcastable or have the same shape. Debugging mismatches here can be a learning curve.
Lastly, I’ll share a go-to operation I perform often: transposing. It flips the array’s shape, making rows into columns and vice versa.
# Transpose of a 2D array
= reshaped_array.T
transposed_array print(transposed_array)
There we have it. The basics are simple enough, right? Once comfortable, these operations become second nature, paving the way to dive deeper into NumPy’s functionality. Keep experimenting and remember, the NumPy documentation (https://numpy.org/doc/stable/reference/) is an excellent resource to further your expertise.
## Array Indexing and Slicing in NumPy
{{< include ../internal/responsive_image4.qmd >}}
Array indexing and slicing are to arrays what grammar is to language: they're essential tools for clear communication. When I first encountered NumPy, I realized how crucial these operations are for efficient numerical computation in Python.
In NumPy, indexing allows you to access individual elements, while slicing lets you access ranges of elements within an array. This flexibility is a game-changer because it helps to work with large datasets without unnecessary loops that slow down the performance.
Here's how simple indexing works:
```python
import numpy as np
# Create a one-dimensional NumPy array
a = np.array([10, 20, 30, 40, 50])
# Access the first element (remember, indexing starts at 0)
print(a[0]) # Output: 10
# Access the last element
print(a[-1]) # Output: 50
Now, let’s say you want to work with a subset of this array. That’s where slicing comes into play.
# Slicing from 1st to 3rd index (4th not included)
print(a[1:4]) # Output: [20 30 40]
# Slicing from the start to the 2nd index
print(a[:3]) # Output: [10 20 30]
# Slicing from the 3rd index to the end
print(a[3:]) # Output: [40 50]
# Slicing with a step - every second element from the whole array
print(a[::2]) # Output: [10 30 50]
That was pretty straightforward for one-dimensional arrays, right? Now, imagine working with multi-dimensional arrays (like matrices in linear algebra). NumPy handles these with the same ease.
# Create a two-dimensional NumPy array
= np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
b
# Access the element on the first row and first column
print(b[0, 0]) # Output: 1
# Access the second row
print(b[1]) # Equivalent to b[1, :] | Output: [4 5 6]
# Access a column - all rows, second column
print(b[:, 1]) # Output: [2 5 8]
# Slicing a sub-matrix - first two rows and first two columns
print(b[:2, :2]) # Output: [[1 2] [4 5]]
Take a moment to play around with indices and slices in the two-dimensional array example above. This ability to pull out parts of an array is immensely powerful, especially when you work with large datasets in scientific computing or machine learning.
One neat trick I’ve learned is using negative indices in slicing. It’s akin to counting backwards from the end of the array:
# Get the last two elements of the first row
print(b[0, -2:]) # Output: [2 3]
You might wonder, why go through all this trouble with indices and slices? Efficiency is the short answer. By leveraging NumPy’s indexing and slicing operations on arrays, your computations eschew superfluous loops and thus gain a significant speedup.
It’s worth noting a subtle yet important detail: slicing creates a “view” of the original array, which means that if you modify the slice, you also modify the original array. This is different from list slicing in plain Python, which creates a copy.
# Slicing creates a view
= a[2:4]
sub_array 0] = -1 # This changes the original array 'a' as well
sub_array[
print(a) # Output: [10 20 -1 40 50]
I encourage you to experiment with indexing and slicing on your own arrays. Create them, play with them, and manipulate them to see firsthand the power of these operations. Remember, practice is key to mastering NumPy’s indexing and slicing, and there’s no substitute for writing your own code to understand these concepts.
And that’s pretty much the gist of array indexing and slicing in NumPy. They might seem rudimentary at first glance, but mastering them will provide a solid foundation for the advanced manipulations we’ll explore in the next sections.
Advanced NumPy Array Manipulations
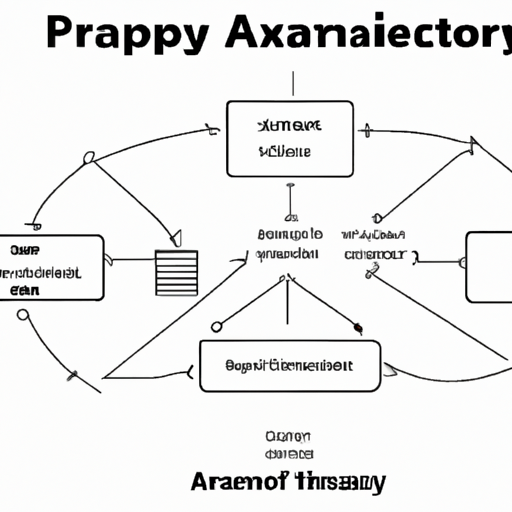
When working with NumPy, a solid understanding of array manipulations can turn complex problems into one-liners. Let’s explore some advanced tricks I use to handle arrays more effectively.
One handy tool is reshape
, which lets you reconfigure an array without changing its data. Imagine having a one-dimensional array of numbers 0 through 11 that you want to structure as a 3x4 grid.
import numpy as np
= np.arange(12)
arr = arr.reshape((3, 4))
grid print(grid)
Broadcasting is another power move. It allows you to perform operations on arrays of different shapes. I wanted to add a fixed value to all elements of a 2D array without looping. Broadcasting made it simple:
= np.ones((3, 3))
arr_2d = arr_2d + 4 # Adds 4 to all elements
addition print(addition)
Sometimes, I need to combine arrays. np.vstack
and np.hstack
quickly become my friends for vertical and horizontal stacking, respectively.
= np.array([1, 2, 3])
a = np.array([4, 5, 6])
b
= np.vstack((a, b))
vstacked = np.hstack((a, b))
hstacked print("Vertical Stack:\n", vstacked)
print("Horizontal Stack:\n", hstacked)
I’ve discovered that data often comes in non-ideal formats. Enter np.concatenate
, a versatile function for joining multiple arrays along any axis. You specify the axis
parameter; default is 0.
= np.concatenate((a.reshape(1,3), b.reshape(1,3)), axis=0)
concatenated print(concatenated)
Flattening is another common task, making a multi-dimensional array one-dimensional. flatten
and ravel
both get the job done, but while flatten
creates a copy, ravel
returns a view (where possible), making it more memory efficient.
= grid.flatten()
flat = grid.ravel()
raveled print("Flattened:", flat)
print("Raveled:", raveled)
But what if we want to apply a function to each element? NumPy’s vectorize
lets us vectorize a custom function, turning it into a function that takes NumPy arrays as input and performs element-wise operations.
def add_if_even(x):
return x + 1 if x % 2 == 0 else x
= np.vectorize(add_if_even)
vectorized_func print(vectorized_func(np.arange(6)))
Let’s not overlook np.split
. When I need to split an array into several smaller arrays, this does the trick. You can specify the number of equal parts or the specific indices where split should occur.
= np.split(np.arange(10), [2, 5])
split_arr print(split_arr)
Occasionally, I run into the need to manipulate array shapes with new axes. np.newaxis
and np.expand_dims
are perfect for increasing the dimensions of your array. They can turn a 1D array into a row or column matrix, which can be pivotal in certain matrix operations.
= a[np.newaxis, :]
newaxis_arr = np.expand_dims(a, axis=1)
expand_dims_arr print("Newaxis result:\n", newaxis_arr)
print("Expand_dims result:\n", expand_dims_arr)
These are just some of the advanced manipulations I utilize with NumPy. They’ve turned tangled messes of loops and logic into clean, readable lines of code. The beauty of NumPy is its simplicity and power—you often find that less is indeed more. As you practice, you’ll discover countless ways to bend NumPy to your will, streamlining your data manipulation and analysis workflows significantly.
NumPy for Linear Algebra and Random Number Generation
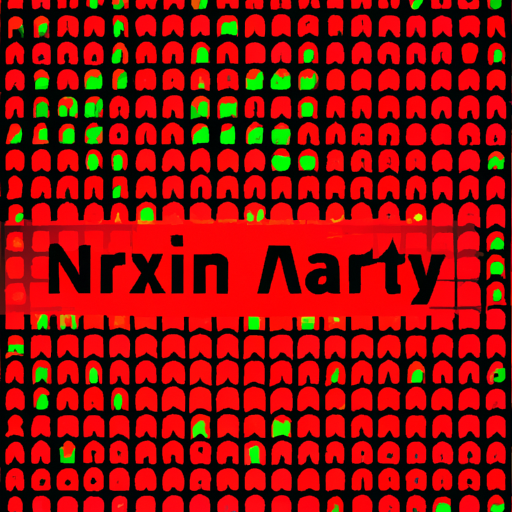
NumPy is the go-to library for numerical computing in Python. What really underscores its utility is how it simplifies tasks in linear algebra and random number generation. Let’s unpack these two aspects.
First up, linear algebra, which is fundamental to so many domains: from data science to engineering. NumPy has a dedicated sub-module, numpy.linalg
, which houses all you need to deal with linear structures efficiently.
For instance, I often find myself dealing with matrices. Creating them in NumPy is intuitive:
import numpy as np
# Creating a 2x2 matrix
= np.array([[1, 2], [3, 4]])
A print(A)
Performing operations like matrix multiplication is equally straightforward. With dot
, you can multiply two arrays.
= np.array([[5, 6], [7, 8]])
B = np.dot(A, B)
product print(product)
Then there’s the matrix inverse, which is crucial and can be a pain to calculate by hand. But NumPy has my back:
= np.linalg.inv(A)
inverse_A print(inverse_A)
Need the determinant? Just one function call away:
= np.linalg.det(A)
det_A print(det_A)
These operations are the tip of the iceberg. Eigenvalues, eigenvectors, solving linear systems – NumPy simplifies all of it.
But wait, there’s more: random number generation – indispensable for simulations, random sampling, and more. NumPy has a numpy.random
module that’s packed with tools.
Generating random numbers is a breeze:
# Generate a random float number between 0 and 1
= np.random.rand()
random_float print(random_float)
Need a bunch of them in an array? No problem:
# Create an array of five random float numbers
= np.random.rand(5)
random_array print(random_array)
What if you’re running an experiment and need reproducibility? Just set a random seed:
42) np.random.seed(
Thereafter, every random number you generate will follow a predictable sequence – essential when you need results that can be duplicated.
NumPy also deals with various distributions. Say you need numbers following a standard normal distribution:
= np.random.randn(5)
normal_array print(normal_array)
Every function is well-documented and just a Google search away. If you’re curious, the NumPy GitHub repository (numpy/numpy) is a rich resource. You can peek into the heart of the functions I’ve used and widen your understanding.
Keep these tools in your arsenal, and you’ll be tackling linear algebra problems and managing random numbers with confidence. Don’t worry too much about memorizing; it’s all about understanding the concepts and knowing where to find the functions when you need them. With practice, it becomes second nature. The beauty of NumPy is it does the heavy lifting, so you can focus on the problem-solving part. Happy computing!
Benchmarking and Best Practices in NumPy
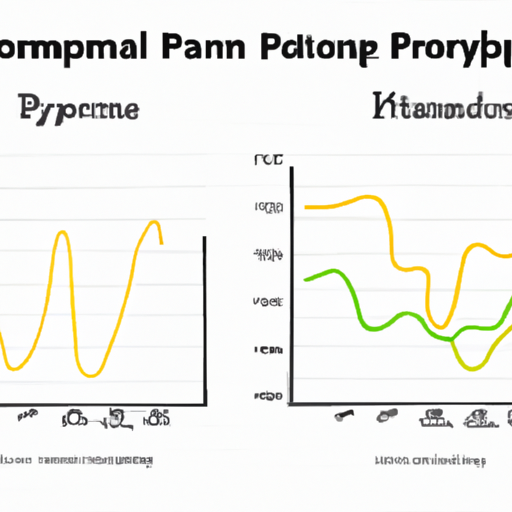
Once you’ve got a handle on the basics of NumPy, it’s time to make sure you’re using it efficiently. I’ve learned that benchmarking and adhering to best practices not only make your code run faster but also make it more readable and maintainable.
Benchmarking is essentially timing how long it takes for your code to run. This is crucial because what you think might be fast might actually be a sluggish piece of code when dealing with large datasets. But before I throw in some benchmarks, let’s make sure you’re following best practices.
I always suggest vectorizing your operations when using NumPy. This means that instead of using loops to process data, you leverage NumPy’s optimized C-based underpinnings. Here’s a simple example comparing the performance of adding two arrays element-wise in a loop versus a vectorized approach:
import numpy as np
import time
# Traditional Python loop
def loop_addition(a, b):
= np.zeros_like(a)
result for i in range(len(a)):
= a[i] + b[i]
result[i] return result
# Vectorized addition in NumPy
def vectorized_addition(a, b):
return a + b
# Initiate arrays
= np.arange(100000)
array1 = np.arange(100000)
array2
# Benchmarking loop_addition
= time.time()
start_time
loop_addition(array1, array2)= time.time()
end_time print(f'Loop time: {end_time - start_time} seconds')
# Benchmarking vectorized_addition
= time.time()
start_time
vectorized_addition(array1, array2)= time.time()
end_time print(f'Vectorized time: {end_time - start_time} seconds')
You’ll notice that the vectorized operation is significantly faster. It utilizes NumPy’s fast array operations and is also easier to read.
Another best practice is to make use of NumPy’s built-in functions whenever possible. NumPy has a wealth of functions that are optimized for array operations. For example, if you want to calculate the mean of an array:
# Calculating mean with a loop
def mean_loop(arr):
= 0
total for num in arr:
+= num
total return total / len(arr)
# NumPy's built-in mean function
= np.random.rand(100000)
array
= time.time()
start_time
mean_loop(array)= time.time()
end_time print(f'Mean loop time: {end_time - start_time} seconds')
= time.time()
start_time
np.mean(array)= time.time()
end_time print(f'Mean NumPy time: {end_time - start_time} seconds')
By comparing the time it takes to calculate the mean using a loop versus using NumPy’s mean
function, you’ll appreciate the optimization that comes out of the box with NumPy.
A small pro tip I’ve picked up: beware of memory usage when copying arrays. NumPy provides copy()
for creating a complete copy of an array but it’s memory-intensive. If you just need a new view of the same data, use array slicing.
Lastly, for beginners eager to dive deeper and find examples of best practices, check out the official NumPy documentation or look through repositories on GitHub. The community often provides excellent examples that can serve as benchmarks for your code.
Keep in mind, tools like timeit
module in Python or Jupyter’s %timeit
magic command provide a more robust way to benchmark code snippets.
Incorporating these suggestions should make your NumPy code not just run smoother but also look neater. Every little bit of performance counts, especially when you are scaling up to larger datasets or complex computations. Happy coding, and keep benchmarking!