Step-by-Step Guide to Sentiment Analysis in Python
Introduction
Sentiment analysis is a fascinating topic. I’ve learned it’s not just about algorithms and code but also about understanding human context. Python allows you to dissect the human context very accurately with the libraries that are available nowadays. In this guide I share my experience with them and provide detailed steps to do so.
Define Your Objective
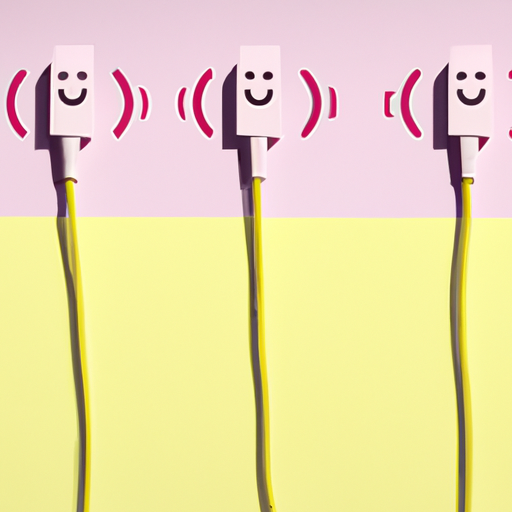
When doing sentiment analysis (in Python or another language), the outcome you’re after is important. Whether it’s gauging public opinion on a new product, monitoring brand reputation, or understanding customer feedback: the clearer the objective, the more targeted and effective the analysis.
Let’s say I want to analyze Twitter data to understand how people feel about a recent tech product launch. This means I’ll be focusing on collecting tweets, preprocessing the text, and categorizing sentiments as positive, negative, or neutral.
Here’s a basic outline in Python of how to do so:
# Define the objective
= "Analyze Twitter sentiment on recent tech product launch."
objective print(f"Objective: {objective}")
Once the objective is spelled out, I can start planning the specifics, like the keywords to track. The code for this would be:
# Set up keywords related to our objective
= ["TechProductName", "TechCompany", "ProductLaunch"]
keywords print(f"Tracking keywords: {keywords}")
With the objective defined and keywords in place, you’re on the right path. But remember, the defined outcome drives the entire analysis process, affecting the choice of tools, algorithms, and even the datasets.
An educational resource that was invaluable to me when I began was Stanford University’s “Natural Language Processing with Deep Learning” course (its 2024 version is available at: https://online.stanford.edu/courses/xcs224n-natural-language-processing-deep-learning). There’s a wealth of material there on sentiment analysis. However, if you’re new to this, focus on understanding the basics before diving deeper.
Now, I like to break down my objective into actionable tasks. For instance:
# Define specific tasks based on the objective
= {
tasks "Data Collection": "Gather tweets using Twitter API",
"Text Preprocessing": "Clean tweets for analysis",
"Model Selection": "Choose a sentiment analysis model appropriate for short texts"
}
for task, description in tasks.items():
print(f"{task}: {description}")
Consider this as mapping the route to your destination. It guides where you need to focus your learning. Should you need a more hands-on approach, GitHub repositories such as sentiment-analysis-python are a treasure trove for practical code snippets and real-world project examples that can offer guidance and inspiration.
From there, it’s all about nailing down the specifics:
# Define more granular objectives
= [
granular_objectives "Learn how to authenticate with Twitter API",
"Understand text cleaning techniques like stopword removal and lemmatization",
"Evaluate different sentiment analysis models like TextBlob and VADER"
]
for index, objective in enumerate(granular_objectives, start=1):
print(f"Step {index}: {objective}")
You’ll have to pair such granular objectives with diligent research. For instance, the VADER tool—a lexicon and rule-based sentiment analysis tool that is specifically attuned to sentiments expressed in social media—can be a great starting point due to its simplicity and effectiveness.
from vaderSentiment.vaderSentiment import SentimentIntensityAnalyzer
# VADER sentiment analysis tool setup for objective
= SentimentIntensityAnalyzer()
analyser
# Example usage
def print_sentiment_scores(sentence):
= analyser.polarity_scores(sentence)
snt print("{:-<40} {}".format(sentence, str(snt)))
# Test it with an example
"The new tech product by TechCompany is revolutionary!") print_sentiment_scores(
The chunk of code above shows you how to employ VADER to examine the sentiment of a single statement. It’s a direct implementation of your goal in the Python universe.
Bear in mind that each project is unique. Never shy away from tweaking tools and methods to bend to your specific purpose. Being flexible and willing to iterate is the name of the game, and it all starts with a crystal-clear objective.
Now, with your objective well-defined and a roadmap at hand, you’re not just coding—you’re setting the stage for actionable insights that can provide real-world value. Happy analysis!
Data Collection
Data collection is pivotal in any sentiment analysis project as it forms the base upon which all the analytical magic happens. I’d like to share how I go about this process with a straightforward and beginner-friendly approach. Just to dive right in, let’s assume we’ve settled on using Twitter as our data source, a gold mine for public opinion.
First off, we need to get access to Twitter’s API. I typically use Tweepy
, a Python library that makes it simple to interact with Twitter’s API. We’ll start by installing it using pip.
pip install tweepy
Once installed, we need to authenticate ourselves with Twitter’s API. You’ll have to create a Twitter developer account and get your API keys: API key
, API secret key
, Access token
, and Access token secret
. Keep these safe!
Here’s how to authenticate your Python script with Tweepy:
import tweepy
# Replace the 'XXXX's with your own keys
= 'XXXX'
api_key = 'XXXX'
api_secret_key = 'XXXX'
access_token = 'XXXX'
access_token_secret
# Setup authentication
= tweepy.OAuthHandler(api_key, api_secret_key)
auth
auth.set_access_token(access_token, access_token_secret)
# Create API object
= tweepy.API(auth) api
Now that we’re authenticated, let’s collect some tweets. For sentiment analysis, we’d want to gather data that’s as relevant as possible to our topic of interest. For example, if we’re investigating public sentiment about the latest iPhone, we’ll probably want to search for tweets mentioning it.
# Define the search term and the date_since date
= "#iPhone -filter:retweets"
search_words = "2023-01-01"
date_since
= tweepy.Cursor(api.search_tweets,
tweets =search_words,
q="en",
lang=date_since).items(5)
since
# Iterate and print tweets
for tweet in tweets:
print(f"{tweet.user.name}: {tweet.text}\n")
With the output from Twitter, we’re not only gathering the text but also user information which might be leveraged later on for more in-depth analysis around demographics.
Lastly, let’s store this data so we can work with it later on. Pandas is a great library to work with such data, so let’s install it and save our tweets to a DataFrame which we then can save to a CSV file.
pip install pandas
After installation, we add the following lines to our code.
import pandas as pd
= [[tweet.user.name, tweet.text] for tweet in tweets]
tweets_list
# Create DataFrame
= pd.DataFrame(tweets_list, columns=['Username', 'Text'])
tweets_df
# Save DataFrame to CSV file
'tweets.csv', index=False) tweets_df.to_csv(
Essentially, what we’ve done here is distilled the process of data collection down to its core Python components: using an API client (Tweepy
), authenticating with an API, querying the API for data, and then storing that data into a useful format (CSV file via Pandas
). This approach is, in a sense, akin to the lean and focused discussions on Hackernews and Reddit where the focus is on practical, executable advice that a beginner can immediately take and implement in their projects.
Remember to abide by Twitter’s API usage policies, respect user privacy, and consider ethical implications while scraping data for sentiment analysis. Happy coding!
Select Sentiment Analysis Tool
Selecting the right tool for sentiment analysis in Python can seem daunting with numerous libraries and APIs at your disposal. I’ve been through a similar dilemma and learned that while choices vary depending on the specific use case, there are some fan favorites in the community that I’d like to share with you.
First, let me introduce you to TextBlob
. It’s a simple library for processing textual data. What I particularly love about TextBlob is its simplicity and how it provides a quick solution to sentiment analysis without much effort. Here’s how you get sentiment scores with TextBlob:
from textblob import TextBlob
= "TextBlob is amazingly simple to use. What great fun!"
text = TextBlob(text)
blob print(blob.sentiment)
You’ll get a polarity score (from -1 to 1) and a subjectivity score (from 0 to 1). Higher polarity indicates a more positive sentiment, while higher subjectivity suggests more personal opinion rather than factual information.
For beginners, TextBlob can feel like magic, but when you’re looking for more robust solutions, NLTK
(Natural Language Toolkit) is a solid choice. It’s a powerful library that’s been around for a while and has a steeper learning curve. To implement sentiment analysis using NLTK’s Vader module, you do the following:
import nltk
from nltk.sentiment.vader import SentimentIntensityAnalyzer
# Download the vader lexicon
'vader_lexicon')
nltk.download(
= "NLTK is quite powerful, but there's a bit of a learning curve."
text = SentimentIntensityAnalyzer()
sid print(sid.polarity_scores(text))
Vader is particularly good for social media text sentiment analysis because it’s been tuned for that sort of informal language.
Now, if you need something cutting-edge, transformers
by Hugging Face is the go-to. It leverages state-of-the-art machine learning models for a wide array of natural language processing (NLP) tasks.
Here’s how you would use a pre-trained model for sentiment analysis:
from transformers import pipeline
# Use the 'sentiment-analysis' pipeline function
= pipeline("sentiment-analysis")
sentiment_pipeline
= "Transformers library offers state-of-the-art models for NLP."
text = sentiment_pipeline(text)[0]
result print(f"label: {result['label']}, with score: {round(result['score'], 4)}")
The Hugging Face model gives you a label (POSITIVE or NEGATIVE) and a confidence score.
Depending on your project scope, you might consider building your model with TensorFlow
or PyTorch
, but training models from scratch requires a good amount of data and computational resources.
Whatever tool you choose, remember that the context of the data matters tremendously in sentiment analysis. The wrong tool for the job could misinterpret the sentiment, and thus, possibly the whole outcome of your analysis. Experiment with these tools and check which one aligns best with your text data.
In short, start simple with TextBlob, move onto NLTK for more control, and consider Hugging Face’s transformers when you’re ready to leverage the power of pre-trained models. Happy analyzing!
Tool Installation
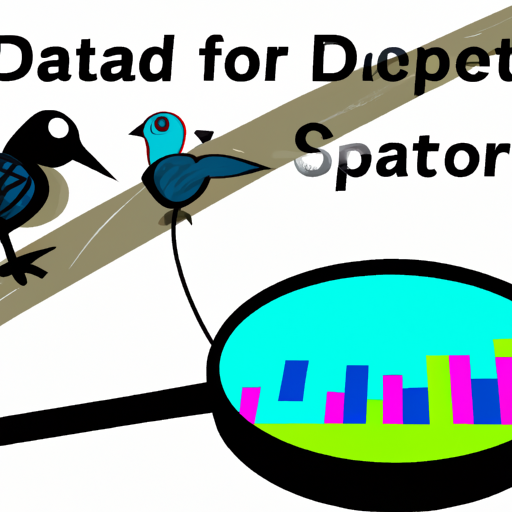
When starting out with sentiment analysis in Python, one of the first hurdles you’ll encounter is setting up the necessary tools. It sounds daunting, but I promise you: with the right resources and a bit of patience, anyone can do it.
Firstly, you’ll need Python installed on your machine. If you haven’t done that yet, head over to the Python downloads page and get the latest version for your operating system. Follow the installation instructions, which typically involve just running the downloaded file and following on-screen prompts.
Now that Python is set up, you’ll need to install specific libraries that make sentiment analysis a breeze. We will primarily use nltk
for natural language processing and textblob
to simplify the analysis process.
I’ll walk you through the installation of these libraries using pip
, which is Python’s package installer. If you don’t have pip
, it’s usually included in the Python installation. If not, you can find the installation guide here.
First, open your command line (Terminal for MacOS/Linux, Command Prompt or PowerShell for Windows) and type:
pip install nltk textblob
After the installation, you need to download some data packages that NLTK will use. Type the following into a Python interpreter or script:
import nltk
'vader_lexicon')
nltk.download('punkt') nltk.download(
The vader_lexicon
is specifically designed for sentiment analysis and is a must-have for our purposes. The punkt
dataset is a tokenizer model that we’ll use later in the preprocessing step.
Next, let’s make sure textblob
is ready to go by running:
from textblob import TextBlob
If you don’t encounter any errors upon importing it, then congratulations, textblob
is successfully installed.
Occasionally, you might need to work with more complex models or datasets that those basic libraries can’t handle. That’s when libraries like tensorflow
or pytorch
come into play, in addition to more advanced NLP libraries like transformers
. However, for the scope of this introduction, nltk
and textblob
will suffice.
To ensure everything is set up correctly, let’s do a quick test. Run the following:
from nltk.sentiment import SentimentIntensityAnalyzer
= SentimentIntensityAnalyzer()
sia = "Python makes machine learning accessible and fun!"
test_sentence print(sia.polarity_scores(test_sentence))
The output should provide you with a dictionary containing the scores for the sentence’s positivity, negativity, neutrality, and compound (an aggregate score). A positive compound score would suggest a positive sentiment, and vice versa for negative sentiments.
Lastly, always remember, maintaining a learning mindset is crucial. If an error pops up, don’t despair. Check out StackOverflow or the generous Python community on Reddit – it’s rich with people ready to help beginners.
With the toolkit now installed, you’re just like a blacksmith with a brand new hammer and anvil, ready to forge ahead. Let’s start analyzing the sentiment of text and uncovering the emotional undertones hidden in plain sight.
Text Preprocessing
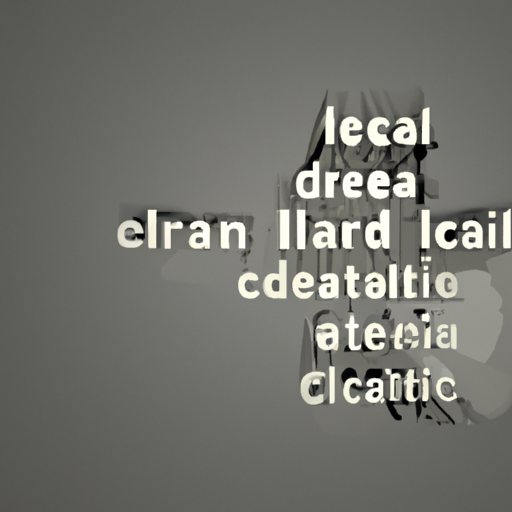
When I first ventured into the realm of sentiment analysis using Python, I realized the importance of clean and structured data. This stage—text preprocessing—is crucial because raw data is often messy and filled with noise. It’s like preparing the canvas before painting; without it, you can’t really showcase the beauty of your analysis.
Let’s dive into the elements of text preprocessing. We’ll start by importing the essential Python libraries.
import re
import string
from nltk.corpus import stopwords
from nltk.stem import PorterStemmer
from nltk.tokenize import word_tokenize
'punkt')
nltk.download('stopwords') nltk.download(
Suppose we have a sample text from a user review:
= "I ordered a coffee at Java Lava Cafe. It was THE worst coffee. Very bad service." sample_text
First off, I would convert the text into lowercase since word capitalization doesn’t affect sentiment.
= sample_text.lower() sample_text
Then, I’d remove any numbers, punctuation, or special characters because they’re irrelevant noise.
= re.sub(r'[\d]', '', sample_text) # Removes digits.
sample_text = sample_text.translate(str.maketrans('', '', string.punctuation)) # Removes punctuation. sample_text
Now, to tokenize the text. Tokenization is chopping it up into pieces, called tokens. Here’s how you take a sentence and split it into words:
= word_tokenize(sample_text) tokens
Stop words like ‘at’, ‘the’, ‘was’, and ‘very’ are typically filtered out because they’re plentiful and carry negligible semantic weight.
= set(stopwords.words('english'))
stop_words = [w for w in tokens if not w in stop_words] filtered_sentence
Sometimes, words have multiple forms (think: “walk”, “walked”, “walking”). Stemming trims words down to their root form.
= PorterStemmer()
stemmer = [stemmer.stem(word) for word in filtered_sentence] stemmed_sentence
Putting it all together:
# Preprocessing function
def preprocess_text(text):
= text.lower()
text = re.sub(r'[\d]', '', text)
text = text.translate(str.maketrans('', '', string.punctuation))
text = word_tokenize(text)
tokens = [w for w in tokens if not w in stopwords.words('english')]
tokens = [stemmer.stem(word) for word in tokens]
tokens return " ".join(tokens)
# Applying the preprocessing
= preprocess_text(sample_text)
clean_text print(clean_text)
Now, the output should be “order coffe java lava cafe worst coffe bad servic” – a cleaner version of the original text suitable for sentiment analysis.
Remember, this is just a foundational step. Depending on the specific requirements of your sentiment analysis and the characteristics of your data, more advanced techniques like lemmatization or handling of negations can be applied.
While we tackled the preprocessing part, always keep in mind that no amount of sophisticated algorithms can outperform the benefits of a well-prepped dataset. It’s the legwork that many neglect, but I assure you, it’s where the magic begins for any sentiment analysis task.
For more in-depth resources, consider checking out Python’s NLTK library documentation or other resources like spaCy, which offer rich text processing capabilities. Additionally, there are countless tutorials and research papers on sentiment analysis that discuss text preprocessing in more detail, accessible through platforms like arXiv or GitHub repositories dedicated to NLP.
Ready to see your algorithms interpret human sentiment? Onwards to tokenization, the next piece of our sentiment analysis puzzle.
Tokenization
In the realm of Sentiment Analysis using Python, one essential step is tokenization. This process involves splitting the text into smaller parts, called tokens, which could be words, phrases, or even sentences. I find it crucial because it helps in structuring the input text in a way that’s manageable for analysis; for instance, identifying keywords that can indicate sentiment polarity.
While there are libraries out there like NLTK and spaCy that can handle tokenization, let me share a straightforward approach using NLTK as it is user-friendly and particularly suitable for beginners. With this library, you can tokenize your text into words or sentences with just a few lines of code.
First, you need to ensure you have the NLTK package installed. If it’s not, install it via pip:
!pip install nltk
Then, you have to import the nltk
library and download the necessary datasets:
import nltk
'punkt') nltk.download(
Now, let’s assume you’ve already collected some data as per the earlier section. Here’s how you proceed with word tokenization. You’ll start with a string of text:
from nltk.tokenize import word_tokenize
= "Natural Language Processing is fascinating."
text = word_tokenize(text)
tokens print(tokens)
You’ll see output like this, which is the list of tokens:
'Natural', 'Language', 'Processing', 'is', 'fascinating', '.'] [
In sentiment analysis, it’s often the case that punctuation and single characters (like the period in our tokens list) aren’t meaningful for determining sentiment. Hence, we might want to get rid of them. Here’s a quick way to filter these out:
= [word for word in tokens if word.isalpha()]
tokens print(tokens)
This filters the list, removing any tokens that don’t consist entirely of alphabetic characters:
'Natural', 'Language', 'Processing', 'is', 'fascinating'] [
I always recommend looking at sentence tokenization as well because sometimes sentiments are better interpreted when you have context that spans across the barriers of mere words into complete sentences. For this task, let’s use the sent_tokenize
function:
from nltk.tokenize import sent_tokenize
= "Natural Language Processing is fascinating. It transforms how we interact with technology."
text = sent_tokenize(text)
sentences print(sentences)
Output:
'Natural Language Processing is fascinating.', 'It transforms how we interact with technology.'] [
When you’re knee-deep in data pre-processing, remember that tokenization is not just a preparatory step; it can significantly influence the accuracy of your sentiment analysis. Well-tokenized text will align better with sentiment lexicons or feed cleaner data into machine learning models.
It’s worth noting that different languages may require special tokenizers to correctly separate words according to their grammatical rules. NLTK supports multilingual tokenization, so you can experiment with non-English texts within the same framework. The nltk.tokenize package offers a variety of tokenizers like PunktTokenizer
for sentence tokenization, which are trained on multiple languages.
Lastly, while NLTK is wonderful for basic NLP tasks and learning, when it comes to scaling up your sentiment analysis pipeline or tackling more complex NLP problems, you might want to explore advanced libraries like spaCy, which offers faster and more sophisticated tokenization processes. But for beginners keen on learning NLP with hands-on practice, NLTK’s simplicity and ease of use provide an excellent starting point.
Model Selection
Model selection involves choosing a suitable algorithm to perform sentiment analysis on the text data you’ve prepared. Given the plethora of models available, this can be an overwhelming step, but I’m going to walk you through a couple of solid starter choices.
Naive Bayes Classifier
A pretty standard starting point is the Naïve Bayes classifier. It’s straightforward, easy to implement, and surprisingly effective for text classification tasks.
from sklearn.naive_bayes import MultinomialNB
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Assuming 'X' is your cleaned, preprocessed text and 'y' are your labels
# (1 for positive sentiment, 0 for negative),
= train_test_split(X, y, test_size=0.2, random_state=42)
X_train, X_test, y_train, y_test
= CountVectorizer()
count_vectorizer = count_vectorizer.fit_transform(X_train)
X_train_counts
# Training the Naive Bayes classifier
= MultinomialNB()
clf
clf.fit(X_train_counts, y_train)
# Test the model performance
= count_vectorizer.transform(X_test)
X_test_counts = clf.predict(X_test_counts)
predictions print(f'Accuracy: {accuracy_score(y_test, predictions)}')
Accuracy here tells us how many labels the model has correctly predicted. For a more robust assessment, you could dig into precision, recall, and F1-score, which help paint a more comprehensive picture of performance across class imbalances.
Support Vector Machine (SVM)
Another model I recommend trying out is Support Vector Machine (SVM). It’s more sophisticated and tends to be highly effective for text classification problems.
from sklearn.svm import SVC
# Your feature extraction remains the same
= train_test_split(X, y, test_size=0.2, random_state=42)
X_train, X_test, y_train, y_test = CountVectorizer()
count_vectorizer = count_vectorizer.fit_transform(X_train)
X_train_counts
# Training the SVM classifier
= SVC()
svm_clf
svm_clf.fit(X_train_counts, y_train)
# Test the model performance
= count_vectorizer.transform(X_test)
X_test_counts = svm_clf.predict(X_test_counts)
predictions print(f'Accuracy: {accuracy_score(y_test, predictions)}')
Training times for SVM can be longer, especially with large datasets, but the results are often worth the wait.
Deep Learning with TensorFlow
For those interested in deep learning, Keras with a TensorFlow backend provides a rich ecosystem for building neural networks.
from keras.models import Sequential
from keras.layers import Dense, Embedding, LSTM
from keras.preprocessing.text import Tokenizer
from keras.preprocessing.sequence import pad_sequences
from sklearn.preprocessing import LabelEncoder
# Tokenization and sequence padding
= Tokenizer(num_words=5000)
tokenizer
tokenizer.fit_on_texts(X)= tokenizer.texts_to_sequences(X)
X_seq = pad_sequences(X_seq, maxlen=200)
X_pad
# Encode our target labels
= LabelEncoder()
label_encoder = label_encoder.fit_transform(y)
y_encoded
# Split the data
= train_test_split(X_pad, y_encoded, test_size=0.2, random_state=42)
X_train, X_test, y_train, y_test
# Build the LSTM model
= Sequential()
model 5000, 128, input_length=X_train.shape[1]))
model.add(Embedding(64, dropout=0.2, recurrent_dropout=0.2))
model.add(LSTM(1, activation='sigmoid'))
model.add(Dense(
compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
model.
# Training the model
=32, epochs=5, validation_data=(X_test, y_test), verbose=2)
model.fit(X_train, y_train, batch_size
# Evaluate the model
= model.evaluate(X_test, y_test, verbose=0)
loss, accuracy print(f'Test Accuracy: {accuracy}')
Deep learning methods require significant computing power and data, but they are unparalleled when it comes to capturing complex patterns in text data.
Remember, each model comes with its own set of assumptions and trade-offs. As a beginner, starting with Naive Bayes and SVM allows you to get familiar with the data and the problem before moving on to more complex models like LSTM.
It’s important to note that no model is universally the best. A part of the model selection process is experimentation to find which model performs best given your specific dataset and problem domain. Good luck with your model selection; may your accuracy soar and your p-values stay low!
Application of Sentiment Analysis
Understanding the general sentiment of customers, users, or any large group of people is invaluable for businesses, organizations, and even on an individual level. Sentiment analysis applications can range from monitoring brand reputation to customer service efficiency, and even to tracking political campaigns. I’ve found that applying sentiment analysis is a captivating journey through natural language processing, machine learning, and human psychology.
When I first embarked upon using sentiment analysis, social media was the prime candidate. Platforms are abundant with user opinions, making them a gold mine for sentiment extraction. With Python, we can scrape data from social platforms and apply sentiment analysis to gauge public opinion.
Here’s an example of how I might analyze Twitter data using Tweepy and TextBlob:
import tweepy
from textblob import TextBlob
# Authenticate to Twitter API (keys masked for privacy)
= 'YOUR_CONSUMER_KEY'
consumer_key = 'YOUR_CONSUMER_SECRET'
consumer_secret = 'YOUR_ACCESS_TOKEN'
access_token = 'YOUR_ACCESS_SECRET'
access_secret
= tweepy.OAuthHandler(consumer_key, consumer_secret)
auth
auth.set_access_token(access_token, access_secret)
= tweepy.API(auth)
api
# Function to perform sentiment analysis
def analyze_sentiment(tweet):
= TextBlob(tweet.text)
analysis return analysis.sentiment
# Search for tweets about a topic
= api.search(q='Python Programming', count=100)
tweets
# Analyze sentiment of each tweet
for tweet in tweets:
= analyze_sentiment(tweet)
sentiment print(f"{tweet.text} Sentiment: {sentiment}")
Another area is customer feedback analysis, which is crucial for improving products and services. You’re not always going to have a clean dataset lying around, so here’s a way to simulate customer reviews and analyze them using Python’s vaderSentiment
:
from vaderSentiment.vaderSentiment import SentimentIntensityAnalyzer
# Simulated customer reviews
= [
reviews "I love this product, it's absolutely wonderful!",
"This is the worst thing I have ever bought.",
"Meh, this was okay, not great but not bad either."
]
= SentimentIntensityAnalyzer()
analyzer
for review in reviews:
= analyzer.polarity_scores(review)
vs print(f"Review: {review}\nSentiment Score: {vs['compound']}\n")
E-commerce sites often use sentiment analysis for product reviews as well. Here, the goal would be to automatically categorize reviews by sentiment, flagging negative ones for customer support follow-up. Again, Python libraries such as nltk
(Natural Language Toolkit) can be incredibly handy:
import nltk
from nltk.sentiment import SentimentIntensityAnalyzer
'vader_lexicon')
nltk.download(
= ["This product had a great performance.", "Do not recommend this at all!", "An average experience, nothing special."]
reviews
= SentimentIntensityAnalyzer()
sia
for review in reviews:
print(review, sia.polarity_scores(review))
Sentiment analysis can also be used in finance to predict market trends based on news headlines or social media sentiment. For instance, using Python’s dataframe manipulation library pandas
and a sentiment analysis library, we can glean insights from financial news:
import pandas as pd
from nltk.sentiment import SentimentIntensityAnalyzer
# Fake news headlines for illustration
= {
data 'headline': [
'Company X stocks soar after positive earnings report',
'Economic downturn fears as market indicators stumble',
'Investors are optimistic about the new tech start-up'
]
}
= pd.DataFrame(data)
df = SentimentIntensityAnalyzer()
sia
'sentiment'] = df['headline'].apply(lambda headline: sia.polarity_scores(headline)['compound'])
df[print(df)
These are just a few possibilities. I’ve discovered that sentiment analysis applications are nearly limitless—from analyzing literary works for emotional arcs to adjusting movie scripts for desired audience emotions. The importance of accurate sentiment analysis can’t be overstated; whether you’re a business gauging brand sentiment, a developer looking to integrate feedback mechanisms into apps, or a researcher understanding social trends, Python’s robust library ecosystem provides the tools needed to transform raw text into meaningful insights.
Results Interpretation
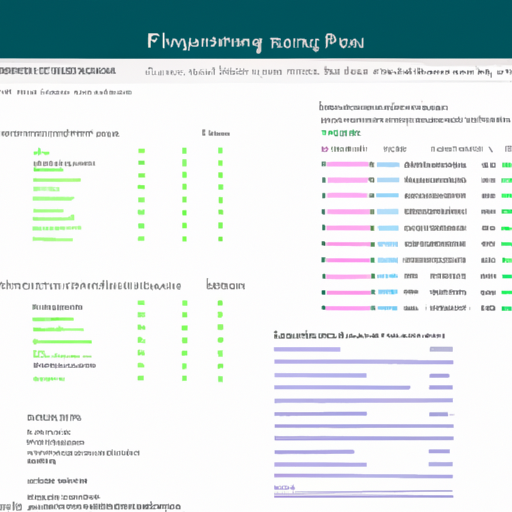
After running sentiment analysis on your dataset, the real gold is found in mining the results for insights. Here’s how I typically interpret the findings.
First, let’s get a visual on the distribution of sentiments. It’s essential to grasp the big-picture trends before delving into specific details.
import matplotlib.pyplot as plt
# Assuming sentiment_scores is a list of sentiment scores from your analysis
def plot_sentiment_distribution(sentiment_scores):
=(10,5))
plt.figure(figsize=50, alpha=0.7)
plt.hist(sentiment_scores, bins'Sentiment Score Distribution')
plt.title('Sentiment Score')
plt.xlabel('Number of Observations')
plt.ylabel(
plt.show()
plot_sentiment_distribution(sentiment_scores)
Looking at the histogram, if you see a normal distribution centered around zero, you can infer that your dataset contains a balance of positive and negative sentiments. A left or right skew could imply a dominance of negative or positive sentiments, respectively.
Next, let’s examine a scatter plot over time to identify sentiment trends. This can illustrate the ebb and flow of sentiment, helpful in contexts such as brand monitoring or tracking public opinion on a topic over time.
import pandas as pd
# Assuming we have a DataFrame df with 'timestamp' and 'sentiment_score' columns
'timestamp'] = pd.to_datetime(df['timestamp'])
df['timestamp', inplace=True)
df.sort_values(
=(15,5))
plt.figure(figsize'timestamp'], df['sentiment_score'], linestyle='solid')
plt.plot_date(df['Sentiment Trend Over Time')
plt.title('Time')
plt.xlabel('Sentiment Score')
plt.ylabel( plt.show()
If there’s a specific event or launch, you’d expect to see a significant shift in sentiment—positively or negatively. Gradual changes could indicate more systemic shifts in perception.
Now, assuming we’ve extracted insights, it’s often instructive to group by themes using the text data itself. We can accomplish simple grouping with pandas as follows.
# Let's group by a hypothetical 'category' column which contains themes from the analysis
= df.groupby('category')['sentiment_score'].mean().sort_values()
grouped_sentiments
='barh', figsize=(10,5))
grouped_sentiments.plot(kind'Average Sentiment Score per Category')
plt.title('Average Sentiment Score')
plt.xlabel('Category')
plt.ylabel( plt.show()
This bar chart informs us which categories (or themes) are received positively or negatively. Such categorization can be derived through additional natural language processing steps, such as topic modeling, assuming you’ve set this up in earlier stages of your analysis.
Real insight comes from diving deeper into specific examples, especially outliers. Let’s pull and examine some sample texts that scored particularly high or low on sentiment.
# Let's examine the most negative sentiments
= df.sort_values('sentiment_score').head(3)
most_negative print("Most Negative Texts:")
print(most_negative[['text', 'sentiment_score']])
# And the most positive
= df.sort_values('sentiment_score', ascending=False).head(3)
most_positive print("\nMost Positive Texts:")
print(most_positive[['text', 'sentiment_score']])
This snippet gives you a tangible view of what constitutes a positive or negative sentiment in your dataset, which can also help in refining your model.
If certain text snippets don’t seem to align with their sentiment scores, you may need to look into further adjusting your model or pre-processing steps. This ties back to the various optional steps in the sentiment analysis pipeline, including ‘Adjustment for Accuracy’.
I should also mention quantifying your model’s error through metrics like accuracy, precision, recall, and F1-score; these aren’t directly part of the results interpretation per se, but they’re relevant to understanding how well your sentiment analysis is performing in a quantifiable way.
Keep in mind that results interpretation is an iterative process—I often go back and tweak some analysis parameters, then re-run and compare._SUPPORT_LINK_PLACEHOLDER_
Optional Visualization
Visualizing data can greatly improve our understanding of the results of sentiment analysis. Even though it’s optional, I often find it immensely beneficial to see the distribution of sentiments across a dataset. There’s something about a well-crafted chart that can communicate insights instantly, which might take several paragraphs to explain in text.
I like to start with the basics: a simple bar chart showing the counts or proportions of each sentiment class. Here’s how you might do it using matplotlib
, the go-to visualization library in Python:
import matplotlib.pyplot as plt
# Assuming `sentiments` is a list of sentiment classes
# e.g., ['positive', 'negative', 'neutral', ...]
= {'positive': sentiments.count('positive'),
sentiment_counts 'negative': sentiments.count('negative'),
'neutral': sentiments.count('neutral')}
plt.bar(sentiment_counts.keys(), sentiment_counts.values())'Sentiment')
plt.xlabel('Count')
plt.ylabel('Sentiment Distribution')
plt.title( plt.show()
Next up, I typically explore more nuanced visualizations like word clouds. A word cloud can show you the most prominent words in your positive, negative, and neutral texts, often revealing patterns you didn’t notice before.
Let’s generate a word cloud for positive sentiment texts:
from wordcloud import WordCloud
# Assuming `positive_texts` is a list of text strings classified as positive
= ' '.join(positive_texts)
positive_text = WordCloud(width=800, height=400, background_color ='white').generate(positive_text)
wordcloud
=(8, 8), facecolor=None)
plt.figure(figsize
plt.imshow(wordcloud)"off")
plt.axis(=0)
plt.tight_layout(pad plt.show()
You might want to dive into how sentiments are spread over time, especially if you’re working with time-stamped data, like tweets. Time-series plots can show trends and patterns in sentiment that could correspond with real-world events.
Here’s an example of how you could visualize sentiment over time:
import pandas as pd
# Assuming `data` is a DataFrame with 'sentiment' and 'date' columns
'date'] = pd.to_datetime(data['date'])
data['date', inplace=True)
data.set_index('sentiment_numeric'] = data['sentiment'].map({'positive': 1, 'negative': -1, 'neutral': 0})
data['D').mean().plot(y='sentiment_numeric', figsize=(10, 6))
data.resample('Date')
plt.xlabel('Average Sentiment Score')
plt.ylabel('Sentiment Over Time')
plt.title( plt.show()
Sometimes, I find it instructive to use scatter plots or density plots to see the distribution of sentiment scores, especially when I am using a sentiment analysis tool that provides a continuous score rather than discrete classes.
Here’s a quick snippet to plot a density plot if you have a scoring system for sentiments:
import seaborn as sns
# Assuming `sentiment_scores` contains continuous sentiment scores
sns.kdeplot(sentiment_scores)'Sentiment Score')
plt.xlabel('Density Plot of Sentiment Scores')
plt.title( plt.show()
Remember, visualizations are a powerful tool but can also be misleading if not used carefully. Always consider the scale, context, and the story behind the data when putting together visualizations. Sometimes a plot may suggest a pattern where there is only random noise, so keep a critical eye and use statistical tools when necessary to support your visual findings.
In the world of sentiment analysis, these plots aren’t just pretty pictures—they’re the bridge between raw data and human insight. Use them wisely, and they can illuminate the narratives hidden in your text.
Adjustment for Accuracy (Optional)
After completing the main steps of sentiment analysis in Python, you might find that your model’s performance isn’t quite where you want it to be. I encountered this myself when I was crunching datasets for analysis. To improve the accuracy, I dug deeper into the fine-tuning process. It’s a balance between art and science. Let’s walk through how to adjust for accuracy in your sentiment analysis models.
Accuracy isn’t just a number; it’s a lens through which the effectiveness of your model’s predictions can be understood. To enhance this, tweaking hyperparameters, using different algorithms, and considering ensemble methods can be valuable.
Adjusting hyperparameters can be a daunting process, but Python’s libraries can simplify this for you. For instance, using GridSearchCV
from sklearn.model_selection
can help you systematically work through multiple combinations.
from sklearn.model_selection import GridSearchCV
from sklearn.linear_model import LogisticRegression
# Example parameters to tune for a Logistic Regression
= {'C': [0.5, 1.0, 10.0],
parameters 'solver': ['newton-cg', 'lbfgs', 'liblinear']}
= LogisticRegression()
model = GridSearchCV(model, parameters)
clf
clf.fit(X_train, y_train)
print(f"Best parameters found: {clf.best_params_}")
X_train
and y_train
should be your training data and labels, respectively. Running the GridSearchCV
can take a while, but you’ll get a fine-tuned model from it.
Sometimes experimenting with different models can lead to better accuracy. For simplistic sentiment analysis, Naive Bayes is commonly used, but I’ve had times when it was outperformed by a Support Vector Machine (SVM). It’s quite straightforward in Python:
from sklearn.svm import SVC
= SVC()
svm_model
svm_model.fit(X_train, y_train)
= svm_model.predict(X_test) svm_predictions
You would simply compare the svm_predictions
to your test labels to examine the model’s accuracy. Don’t forget to use proper performance metrics, such as the accuracy_score
from sklearn.metrics
to evaluate your model.
Ensemble methods combine predictions from various models. Using VotingClassifier
from sklearn.ensemble
can improve accuracy, as different models capture different patterns in the data.
from sklearn.ensemble import RandomForestClassifier, VotingClassifier
from sklearn.naive_bayes import MultinomialNB
# Create different models to ensemble
= RandomForestClassifier(n_estimators=50, random_state=1)
rf_clf = MultinomialNB()
nb_clf
# Combine them in a Voting Classifier
= VotingClassifier(
voting_clf =[('rf', rf_clf), ('nb', nb_clf)],
estimators='soft'
voting
)
voting_clf.fit(X_train, y_train)
= voting_clf.predict(X_test) ensemble_predictions
It’s crucial to note that accuracy isn’t the end-all metric, especially in skewed datasets. It’s important to check precision, recall, and the F1 score as well.
from sklearn.metrics import classification_report
= classification_report(y_test, ensemble_predictions)
report print(report)
This will give you a detailed report of your model’s performance across multiple metrics. The F1 score, in particular, is a better measure of the test’s accuracy, as it considers both the precision and the recall of the test.
Remember that constantly tweaking and testing is how any analyst iterates towards the most accurate model. I’ve learned that while patience may be a virtue, perseverance is a necessity in the field of machine learning and sentiment analysis. Keep refining, keep evaluating, and most importantly, don’t be afraid to experiment with new approaches, because even a small adjustment can lead to significant improvements in accuracy.
Deployment (Optional)
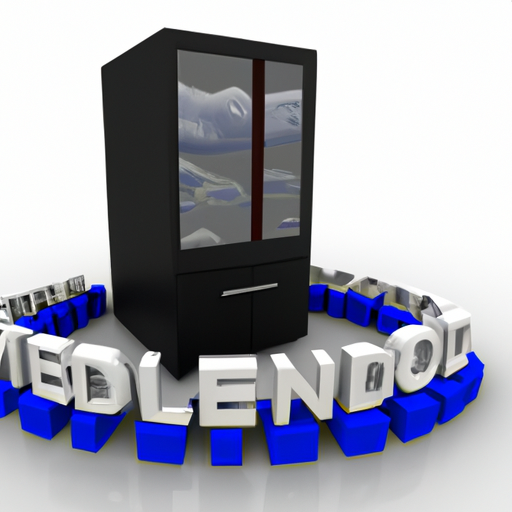
Once you’ve traversed the journey of understanding and applying sentiment analysis in Python, the next exhilarating step could be deploying your model. By doing so, you make your hard work accessible and usable to others or integrate it into an existing system. I’ll guide you through an example of deploying a sentiment analysis model using Flask, a Python micro web framework.
Flask is lightweight and easy to use, making it a great choice for setting up a simple web service. First, ensure you’ve installed Flask using pip:
pip install Flask
With Flask installed, let’s craft a simple web server to serve our sentiment analysis model. Create a file named app.py
and open it in your editor of choice. Here’s a basic skeleton of what your app.py
might look like:
from flask import Flask, request, jsonify
from my_sentiment_model import analyze_sentiment
= Flask(__name__)
app
@app.route('/analyze', methods=['POST'])
def analyze():
# Extract text from the request
= request.get_json(force=True)
data = data['text']
text
# Use the model to analyze sentiment
= analyze_sentiment(text)
result
# Return the result as a JSON response
return jsonify(result)
if __name__ == '__main__':
=True) app.run(debug
In the code above, replace my_sentiment_model
with the module name where your sentiment analysis function analyze_sentiment
is defined. This function should take a string of text as input and return the sentiment analysis results.
To deploy this web service, simply run the app.py
script:
python app.py
Your Flask app will start running on http://127.0.0.1:5000 by default.
Now, to test your deployment, you could use a tool like curl
or Postman to send a POST request with some text to your /analyze
route and see if you get the expected sentiment analysis result. Here’s how you might do this with curl from the command-line:
curl -X POST -H "Content-Type: application/json" \
-d '{"text":"I love coding in Python!"}' \
http://127.0.0.1:5000/analyze
This would return a JSON response from your Flask app with the sentiment analysis result. It’s quite a marvel to see your model handling real requests!
Although this is a bare-bones deployment example, for production you’d need to consider more like security, scaling, and handling failure cases. You might deploy your Flask app using a more robust server like Gunicorn and even containerize your app using Docker.
A few lines of code and you’ve gone from training models on your local machine to serving them as a web API. Isn’t that quite empowering?
Remember, deployment architecture can get complex depending on the scale and there are other approaches such as using serverless functions on AWS Lambda or deploying on platforms like Heroku.
For more advanced users, you can explore these areas further, but for now, you’ve crossed a major bridge in making your sentiment analysis model useful in the real world. Keep tinkering, and keep learning – the world of machine learning is vast and ever-evolving.
Monitoring and Updates (Optional)
Monitoring and updating your sentiment analysis models is as crucial as the initial deployment. I’ve learned through trial and error that what works today may not work as effectively tomorrow. Language evolves, and so does the context within which words are used. This is true whether you’re analyzing tweets, product reviews, or any other text data.
Here’s what I usually do to keep my models fresh and relevant:
Continuous Monitoring
It’s important to consistently track the performance of your sentiment analysis models. To make this task manageable, I set up a simple logging system that records predictions and actuals. Here’s a basic example of how you might log your model’s output using Python’s logging module:
import logging
# Configure logging
='sentiment_analysis.log', level=logging.INFO)
logging.basicConfig(filename
def log_predictions(data, predictions):
for input_text, prediction in zip(data, predictions):
f"Text: {input_text[:50]}... | Prediction: {prediction}") logging.info(
This logs the text being analyzed (trimmed to 50 characters for brevity) and the model’s prediction. Over time, you will accumulate a log that can help you identify patterns or degradation in performance.
Regular Model Updates
If you notice that the model’s performance is starting to wane, it might be time for an update. I often retrain my models on a mix of the old data and new, fresh examples that reflect the latest use of language. You don’t have to start from scratch—simply update your existing model. Here’s how you might append new training data and update your model using scikit-learn:
from sklearn.externals import joblib
from sklearn.svm import SVC
# Load the existing model
= joblib.load('sentiment_model.pkl')
model
# Your new training data
= get_new_data()
X_new_data, y_new_data
# Add the new data to the old (ensure your data is preprocessed in the same way)
= np.concatenate((X_old, X_new_data))
X_combined = np.concatenate((y_old, y_new_data))
y_combined
# Retrain the model
model.fit(X_combined, y_combined)
# Save the updated model
'sentiment_model_updated.pkl') joblib.dump(model,
Leveraging the Community
Lastly, the community can be an invaluable resource. Platforms like GitHub are teeming with people working on similar problems. I often browse repositories for the latest updates in sentiment analysis or look for new models that people are discussing on forums like Hackernews or Reddit.
Here’s an example of how you could clone a GitHub repo that has an updated sentiment analysis model which you might consider using:
git clone https://github.com/username/sentiment-analysis-model.git
cd sentiment-analysis-model
# Let's say the repo has a Python script to load and apply the model
from new_model import UpdatedModel
# Load the new model
= UpdatedModel.load_model('path/to/new_model')
model
# Apply this model to your data
= model.predict(X_data) predictions
Then, integrate these predictions into your logging system as demonstrated earlier, and you’re good to go. Good luck!