Building a Sentiment Analysis Model in Python (2023)
Basic Sentiment Analysis in Python
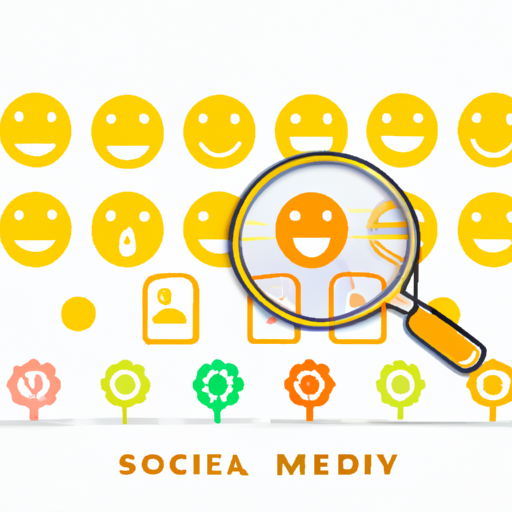
Sentiment analysis has become a quintessential part of understanding the vast array of text data generated in the modern digital world. In essence, it’s a method used to evaluate the tone of written text - is the author’s intention positive, negative, or neutral? As a Python enthusiast, I love diving into this topic, because Python’s natural language processing (NLP) tools make sentiment analysis fairly accessible.
Let me start by jumping straight into practical Python code, which is how I learn best. Before engaging in complex model building, it’s crucial to grasp the basics of sentiment analysis. I’ll guide you through the initial steps of preprocessing text data for sentiment analysis. Yes, there are more extensive parts of the process, such as setting up an environment or evaluating a model, but here we’re focusing on the crux of sentiment analysis.
First, we need a dataset to work with. For simplicity, let’s consider a small sample of sentences:
= ["I love Python programming!",
sentences "I hate when my code breaks.",
"Programming can be challenging yet rewarding."]
Now, to analyze these sentiments, we’ll use the popular nltk
library. If it’s not already present in your Python environment, you can quickly install it using pip install nltk
. One of the simplest sentiment analysis tools in nltk
is the Vader
module, which is built specifically for analyzing sentiments in social media texts.
from nltk.sentiment import SentimentIntensityAnalyzer
def analyze_sentiments(sentences):
= SentimentIntensityAnalyzer()
sia for sentence in sentences:
= sia.polarity_scores(sentence)
sentiment_score yield sentence, sentiment_score
When you run the analyze_sentiments
function on our sentences
, it will churn out a polarity score for each one. The score outputs a dictionary containing “neg”, “neu”, “pos”, and “compound” values, signifying negative, neutral, positive, and a combined score respectively.
for sentence, score in analyze_sentiments(sentences):
print(f"Sentence: {sentence}\nScore: {score}\n")
As a beginner, you might be wondering about the compound score. It’s a metric that calculates the sum of all the lexicon ratings and normalizes them between -1 for most extreme negative and +1 for most extreme positive.
Now, beyond basic libraries, Python offers extensive frameworks for NLP tasks such as TextBlob
. One of the reasons I prefer it for beginner-friendly sentiment analysis is its straightforward approach and ease of use.
from textblob import TextBlob
for sentence in sentences:
= TextBlob(sentence)
testimonial print(f"Sentence: {sentence}\nPolarity: {testimonial.sentiment.polarity}\n")
The above code introduces us to the TextBlob
object, which possesses a property called sentiment. This in turn has an attribute polarity
that ranges from -1 to 1. The TextBlob
library internally employs pattern analysis and text processing to provide this functionality.
Though these tools give us a quick dive into sentiment analysis, ultimately, to harness the full power of sentiment interpretation in Python, we’ll need to build and train more robust models using machine learning libraries like scikit-learn or deep learning frameworks like TensorFlow. But these are topics for another section, such as when we discuss Step-by-Step Guide to Sentiment Analysis in Python.
Remember, don’t be distressed if your initial foray doesn’t immediately yield high-accuracy results. Real-world text data is messy, and sentiment can be subtle and complex. But that’s the beauty of NLP – there’s always more to learn and improve. Keep tinkering with these tools and datasets, and you’ll soon start seeing the world of text data in a whole new light.
Setting Up Your Python Environment
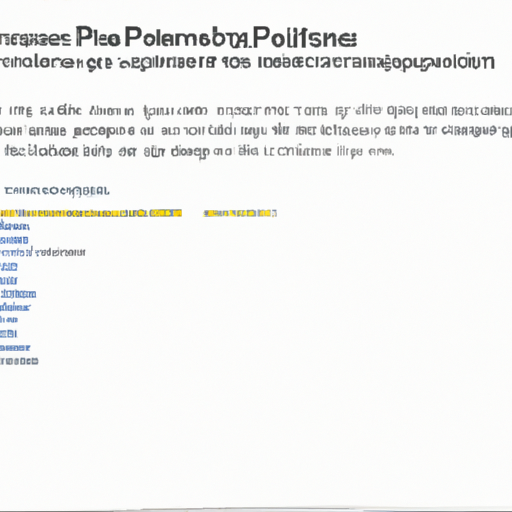
Setting up your Python environment is the bedrock of any coding journey, especially when delving into the world of sentiment analysis. I remember the days when I first dipped my toes into Python; it was a mixed bag of excitement and bewilderment. So, I’ll walk you through the steps using my experience as a compass to get you started smoothly.
Before writing a single line of code for sentiment analysis, let’s make sure we have Python installed. If you don’t have it yet, go to the official Python website and download the version appropriate for your operating system. I usually run the latest stable release, because let’s face it, who doesn’t like the freshest features?
Once you have Python up and running, the next step is to install pip, Python’s package installer. If you’re interested in applying Python for data analysis with multiple cores, consider looking into Dask. A lot of the time, pip comes with Python. You can check this by running the following command in your terminal:
-m ensurepip --upgrade python
With pip installed, setting up an isolated environment for our sentiment analysis project is crucial. We don’t want package conflicts messing up our day, do we? For this purpose, I love using virtualenv
. Simply install it using pip:
pip install virtualenv
Now that virtualenv
is ready, create a new directory for your project and navigate to it in your terminal. Then, create a virtual environment within the project directory:
virtualenv sentiment_env
Activating the virtual environment is different on Windows versus Unix-based systems. On Unix-based systems, you’d run:
source sentiment_env/bin/activate
On Windows, it’s a bit different:
sentiment_env\Scripts\activate
The crucial libraries for sentiment analysis you’re going to need are nltk
for natural language processing tasks and pandas
for handling data structures. Trust me, they’re lifesavers. Install them using pip:
pip install nltk pandas
To get you started on how we use these libraries a bit, let me show you a quick nltk
setup. You’ll want to download the stopwords package for filtering out those pesky common words that carry no sentiment value:
import nltk
'stopwords') nltk.download(
One more library I swear by is matplotlib
, as visualizing data is always enlightening. Installing it is as easy as pie:
pip install matplotlib
To test that everything is in working order, try this simple code to visualize the frequency distribution of words in a text:
import matplotlib.pyplot as plt
from nltk import FreqDist
from nltk.tokenize import word_tokenize
= "Python is amazing. It's easy to understand and effective at tasks."
sample_text = word_tokenize(sample_text)
tokens
= FreqDist(tokens)
frequency_distribution 30, cumulative=False)
frequency_distribution.plot( plt.show()
You should see a nifty graph pop up illustrating the frequency of each word in your sample_text
. Voilà, you’re now on the path to mastering sentiment analysis in Python.
Don’t hesitate to dive into the vast ocean of Python documentation and resources provided by various universities or collaborative projects hosted on platforms like GitHub. I find checking out repositories related to sentiment analysis, such as TextBlob, often offers some real-world code insights and inspiration.
Working with Text Data in Python
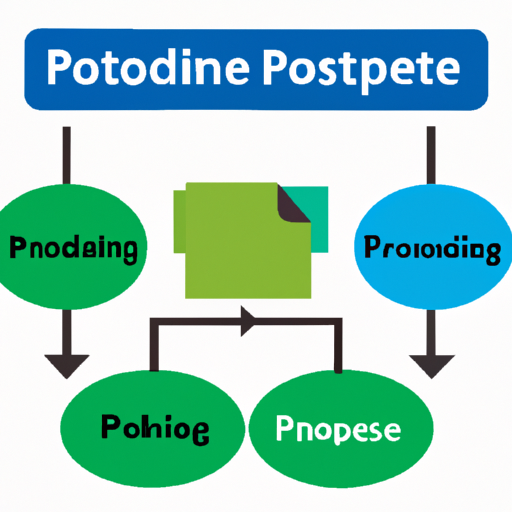
Working with text data in Python is at the very heart of sentiment analysis. For anyone beginning this journey, grappling with strings, text files, and processing techniques is essential. When I first got my hands on natural language processing (NLP), it became clear that Python, with its rich set of libraries, was the sanctuary for data scientists and hobbyists alike.
First things first, Python’s built-in string methods are like the Swiss Army knife for any text manipulation task, making life much simpler. Here’s a crash course in string operations:
= "Machine learning is fascinating!"
text print(text.lower()) # Lowercase: "machine learning is fascinating!"
print(text.upper()) # Uppercase: "MACHINE LEARNING IS FASCINATING!"
print(text.replace("fascinating", "awesome")) # Replace words
Now, in the context of sentiment analysis, cleaning and preparing the text data is crucial. Common preprocessing steps include tokenization, removing stopwords, and stemming. For these, the nltk
library—a power pack of NLP tools—comes to the rescue. If you haven’t already, installing it is super easy:
!pip install nltk
Let’s tokenize a sentence, which means splitting it into individual words, or “tokens”:
import nltk
from nltk.tokenize import word_tokenize
'punkt') # Necessary for tokenization
nltk.download(
= "Python empowers data analysis."
sentence = word_tokenize(sentence)
tokens print(tokens) # ['Python', 'empowers', 'data', 'analysis', '.']
But textual data is often messy, filled with common words like “the”, “is”, “in” which, while necessary for sentence construction, don’t add much value for analysis. These are stopwords, and we usually remove them; similar to how cleaning up the data is pivotal in machine learning as discussed in our article on Cleaning Up the Data Mess: The Real Hero in Machine Learning.
from nltk.corpus import stopwords
'stopwords')
nltk.download(= set(stopwords.words('english'))
stop_words
= [word for word in tokens if not word.lower() in stop_words]
filtered_tokens print(filtered_tokens) # ['Python', 'empowers', 'data', 'analysis', '.']
For sentiment analysis, it’s vital to understand the root of words. This process, called stemming, chops off word suffixes to retrieve the base or stem of the word. Here’s how one can do it using nltk
:
from nltk.stem import PorterStemmer
= PorterStemmer()
stemmer = [stemmer.stem(word) for word in filtered_tokens]
stemmed_words print(stemmed_words) # ['python', 'empow', 'data', 'analysi', '.']
However, sometimes stemming is too crude, and something more sophisticated, like lemmatization, is required. It delivers the base or dictionary form of a word, known as the lemma:
'wordnet')
nltk.download(from nltk.stem import WordNetLemmatizer
= WordNetLemmatizer()
lemmatizer = [lemmatizer.lemmatize(word) for word in filtered_tokens]
lemmatized_words print(lemmatized_words) # ['Python', 'empowers', 'data', 'analysis', '.']
The beauty of lemmatization is that convert words like “wolves” to “wolf”, which is more informative than just chopping off to “wolv” as stemming might do.
After pre-processing, you’ll need to convert text data to numerical form (since ML algorithms thrive on numbers). One popular method is the Bag-of-Words (BoW) model, which represents text as an unordered collection of words. Let’s quickly whip up a BoW model using scikit-learn:
from sklearn.feature_extraction.text import CountVectorizer
= ["Python is awesome", "Machine learning is cool", "I love NLP"]
docs = CountVectorizer()
vectorizer = vectorizer.fit_transform(docs)
bow
print(bow.toarray()) # BoW representation: [[1 0 1 0 0 1],[0 1 0 0 1 1],[0 0 0 1 1 0]]
print(vectorizer.get_feature_names_out()) # ['awesome', 'cool', 'is', 'love', 'learning', 'python']
While this captures frequency, it lacks context and identifying which words are more “important”. Hence, Term Frequency-Inverse Document Frequency (TF-IDF) takes the stage:
from sklearn.feature_extraction.text import TfidfVectorizer
= TfidfVectorizer()
tfidf_vectorizer = tfidf_vectorizer.fit_transform(docs)
tfidf
print(tfidf.toarray()) # TF-IDF representation
print(tfidf_vectorizer.get_feature_names_out()) # ['awesome', 'cool', 'is', 'love', 'learning', 'python']
In TF-IDF, words that appear frequently across many documents will be penalized, and unique words will get a boost, being more representative of the document’s content.
Armed with these text processing tools, you’re now set to edge closer to sentiment analysis. Always remember, different projects require different preprocessing steps. Experimentation is key, and Python’s flexibility allows just that. Dive in, fine-tune, and watch how your models achieve better accuracy with cleaner, sharper data!
Building and Training a Sentiment Analysis Model
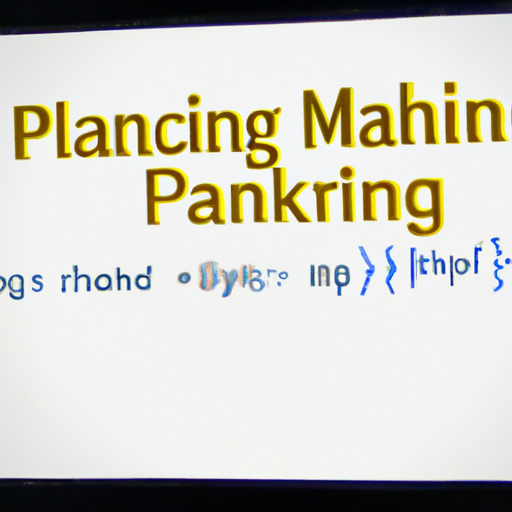
Building and training a sentiment analysis model can seem daunting at first, but with the right tools and understanding, it becomes an approachable task. In my experience, a step-by-step approach helps demystify the process. Let’s dive into how this is done in Python.
Sentiment analysis models typically classify text into categories like positive, negative, or neutral sentiments. To build such a model, I use machine learning libraries such as scikit-learn and nltk, although there are many other options out there.
Firstly, you’ll need a dataset. A popular one to start with is the IMDB dataset that contains movie reviews, as it has training and test data neatly split for us already. You can get the dataset quickly with the help of nltk:
import nltk
'movie_reviews')
nltk.download(from nltk.corpus import movie_reviews
Before training, the data has to be prepared. Start by loading the reviews and their respective sentiments:
= [(list(movie_reviews.words(fileid)), category)
documents for category in movie_reviews.categories()
for fileid in movie_reviews.fileids(category)]
The next step is feature extraction. I typically use the Bag of Words model, which converts text documents into numerical feature vectors:
from sklearn.feature_extraction.text import CountVectorizer
# Joining the individual words back into strings
= [(" ".join(document), category) for document, category in documents]
documents
# Splitting the data into two lists
= zip(*documents)
reviews, sentiments
# Creating the feature vector
= CountVectorizer()
vectorizer = vectorizer.fit_transform(reviews) features
With our features ready, let’s split the data into training and test sets using scikit-learn:
from sklearn.model_selection import train_test_split
= train_test_split(
X_train, X_test, y_train, y_test =0.2, random_state=42) features, sentiments, test_size
Now, pick a machine learning model to start training. A good beginning choice is the Naive Bayes classifier. It’s simple and often effective for text classification:
from sklearn.naive_bayes import MultinomialNB
# Train the model
= MultinomialNB()
model model.fit(X_train, y_train)
After training the model, it’s important to test its accuracy:
# Test the model
print(f"Model Accuracy: {model.score(X_test, y_test)*100:.2f}%")
At this point, you’ve got a basic sentiment analysis model up and running! But there’s always room for improvement. For instance, you could experiment with different feature extraction techniques like TF-IDF, or try out different machine learning algorithms.
One aspect I found crucial while working on similar projects is fine-tuning the model using grid search to optimize its hyperparameters, which significantly influences performance:
from sklearn.model_selection import GridSearchCV
# Define the parameter grid
= {'alpha': [0.1, 1, 5, 10]}
param_grid
# Instantiate grid search
= GridSearchCV(MultinomialNB(), param_grid, cv=5)
grid
# Fit the model
grid.fit(X_train, y_train)
# Best model
= grid.best_estimator_
best_model print(f"Best model accuracy: {best_model.score(X_test, y_test)*100:.2f}%")
Remember, sentiment analysis models can always be refined by adding more data, employing more complex algorithms such as deep learning, or using advanced natural language processing techniques, similar to how Polars can be used for fast data analysis in Python. The field is vast, and these first steps are just the beginning of a journey into text analysis. Keep experimenting, and you’ll be on your way to crafting a robust sentiment analysis model.
Evaluating and Improving Your Model
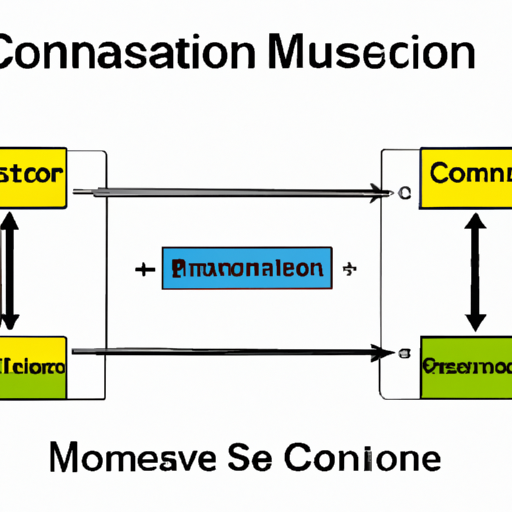
Once you’ve built and trained your sentiment analysis model, you might feel like you’re done. But there’s an important step left that can significantly improve your model’s performance: evaluation and improvement. It’s crucial to assess how well your model is doing and then take steps to enhance its accuracy.
I remember the initial models I built; they seemed great until I rigorously tested them. During the evaluation phase, you’ll often find overlooked data quirks, overfitting, or that your model doesn’t generalize well to new data. That’s why I always allocate substantial time for model evaluation and iterative improvement now.
Let’s dive into ways to evaluate your sentiment analysis model using Python.
First, you need an evaluation metric. Accuracy is the most straightforward metric, reflecting the proportion of correct predictions made by your model out of all predictions. However, depending on your dataset and the balance of classes (positive, negative, neutral), you might want to consider precision, recall, and F1-score as well.
from sklearn.metrics import accuracy_score, classification_report
# Assume y_true are your true labels and y_pred are your model's predictions
= [...]
y_true = [...]
y_pred
print(f"Accuracy: {accuracy_score(y_true, y_pred)}")
print(classification_report(y_true, y_pred))
However, the classification report and confusion matrix will give you much richer insights. They show you how your model performs on each class and flag potential biases—for instance, if it’s great at identifying positive tweets but poor with negative ones.
from sklearn.metrics import confusion_matrix
import seaborn as sns
import matplotlib.pyplot as plt
= confusion_matrix(y_true, y_pred)
conf_matrix =True, fmt='g')
sns.heatmap(conf_matrix, annot'Predicted labels')
plt.xlabel('True labels')
plt.ylabel( plt.show()
After evaluation, tweaking your model is the next step. One common improvement strategy is hyperparameter tuning. I’ve often found that changing the model’s parameters—like learning rate, number of layers, or size of layers—can have a big impact on performance.
from sklearn.model_selection import GridSearchCV
# Model is your sentiment analysis model, parameters is a dictionary of params you want to tune
= {
parameters 'vect__ngram_range': [(1, 1), (1, 2)],
'tfidf__use_idf': (True, False),
# ... add more grid search parameters here
}
= GridSearchCV(estimator=model, param_grid=parameters, n_jobs=-1, cv=5)
grid_search
grid_search.fit(X_train, y_train)
print(f"Best Score: {grid_search.best_score_}")
print(f"Best Params: {grid_search.best_params_}")
Another key aspect is feature engineering. You might enhance your performance significantly by including new features such as:
- Text length
- Use of emojis
- Capitalization patterns
Adding these features is straightforward:
import pandas as pd
import emoji
# Assume df is your DataFrame containing the text column `tweet`
'text_length'] = df['tweet'].apply(len)
df['emoji_count'] = df['tweet'].apply(lambda x: len([c for c in x if c in emoji.UNICODE_EMOJI]))
df['capital_ratio'] = df['tweet'].apply(lambda x: sum(1 for c in x if c.isupper()) / len(x)) df[
Lastly, don’t forget about your dataset. More data, cleaning noisy labels, or correcting class imbalances can also lead to a better model. Always iterate on both your data and model—it’s a symbiotic process where both need attention to achieve the best results.
In conclusion, building a sentiment analysis model is just the beginning. Evaluation and improvement are where you refine your skills and craft a truly reliable and effective tool. Always iterate, always test with new data, and stay curious about potential improvements. This transformation from raw model to polished product is not only critical but also one of the most exciting parts of a data scientist’s work.